mirror of
https://github.com/vim-airline/vim-airline
synced 2025-02-20 12:36:58 +00:00
Merge pull request #1788 from hallettj/extension/languageclient
Extension to report errors and warnings from LanguageClient plugin
This commit is contained in:
commit
f5676d1fa1
@ -4,6 +4,10 @@ This is the Changelog for the vim-airline project.
|
||||
|
||||
## [Unreleased]
|
||||
|
||||
- New features
|
||||
- Extensions:
|
||||
- [LanguageClient](https://github.com/autozimu/LanguageClient-neovim)
|
||||
|
||||
## [0.9] - 2018-01-15
|
||||
- Changes
|
||||
- Look of default Airline Symbols has been improved [#1397](https://github.com/vim-airline/vim-airline/issues/1397)
|
||||
|
@ -185,6 +185,9 @@ vim-airline integrates with a variety of plugins out of the box. These extensio
|
||||
#### [localsearch][54]
|
||||

|
||||
|
||||
#### [LanguageClient][57]
|
||||
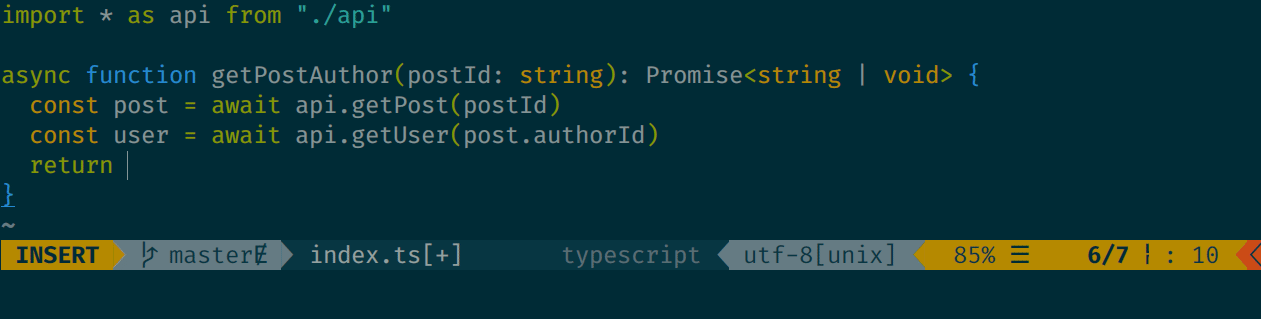
|
||||
|
||||
## Extras
|
||||
|
||||
vim-airline also supplies some supplementary stand-alone extensions. In addition to the tabline extension mentioned earlier, there is also:
|
||||
@ -340,3 +343,4 @@ MIT License. Copyright (c) 2013-2017 Bailey Ling & Contributors.
|
||||
[54]: https://github.com/mox-mox/vim-localsearch
|
||||
[55]: https://github.com/k-takata/minpac/
|
||||
[56]: https://github.com/vim-airline/vim-airline-themes/blob/master/autoload/airline/themes/dark_minimal.vim
|
||||
[57]: https://github.com/autozimu/LanguageClient-neovim
|
||||
|
@ -254,6 +254,11 @@ function! airline#extensions#load()
|
||||
call add(loaded_ext, 'ale')
|
||||
endif
|
||||
|
||||
if (get(g:, 'airline#extensions#languageclient#enabled', 1) && exists(':LanguageClientStart'))
|
||||
call airline#extensions#languageclient#init(s:ext)
|
||||
call add(loaded_ext, 'languageclient')
|
||||
endif
|
||||
|
||||
if get(g:, 'airline#extensions#whitespace#enabled', 1)
|
||||
call airline#extensions#whitespace#init(s:ext)
|
||||
call add(loaded_ext, 'whitespace')
|
||||
|
101
autoload/airline/extensions/languageclient.vim
Normal file
101
autoload/airline/extensions/languageclient.vim
Normal file
@ -0,0 +1,101 @@
|
||||
" MIT License. Copyright (c) 2013-2018 Bjorn Neergaard, w0rp, hallettj et al.
|
||||
" vim: et ts=2 sts=2 sw=2
|
||||
|
||||
scriptencoding utf-8
|
||||
|
||||
let s:error_symbol = get(g:, 'airline#extensions#languageclient#error_symbol', 'E:')
|
||||
let s:warning_symbol = get(g:, 'airline#extensions#languageclient#warning_symbol', 'W:')
|
||||
let s:show_line_numbers = get(g:, 'airline#extensions#languageclient#show_line_numbers', 1)
|
||||
|
||||
" Severity codes from the LSP spec
|
||||
let s:severity_error = 1
|
||||
let s:severity_warning = 2
|
||||
let s:severity_info = 3
|
||||
let s:severity_hint = 4
|
||||
|
||||
" After each LanguageClient state change `s:diagnostics` will be populated with
|
||||
" a map from file names to lists of errors, warnings, informational messages,
|
||||
" and hints.
|
||||
let s:diagnostics = {}
|
||||
|
||||
function! s:languageclient_refresh()
|
||||
if get(g:, 'airline_skip_empty_sections', 0)
|
||||
exe ':AirlineRefresh'
|
||||
endif
|
||||
endfunction
|
||||
|
||||
function! s:record_diagnostics(state)
|
||||
let result = json_decode(a:state.result)
|
||||
let s:diagnostics = result.diagnostics
|
||||
call s:languageclient_refresh()
|
||||
endfunction
|
||||
|
||||
function! s:get_diagnostics()
|
||||
call LanguageClient#getState(function("s:record_diagnostics"))
|
||||
endfunction
|
||||
|
||||
function! s:diagnostics_for_buffer()
|
||||
return get(s:diagnostics, expand('%:p'), [])
|
||||
endfunction
|
||||
|
||||
function! s:airline_languageclient_count(cnt, symbol)
|
||||
return a:cnt ? a:symbol. a:cnt : ''
|
||||
endfunction
|
||||
|
||||
function! s:airline_languageclient_get_line_number(type) abort
|
||||
let linenumber_of_first_problem = 0
|
||||
for d in s:diagnostics_for_buffer()
|
||||
if d.severity == a:type
|
||||
let linenumber_of_first_problem = d.range.start.line
|
||||
break
|
||||
endif
|
||||
endfor
|
||||
|
||||
if linenumber_of_first_problem == 0
|
||||
return ''
|
||||
endif
|
||||
|
||||
let open_lnum_symbol = get(g:, 'airline#extensions#languageclient#open_lnum_symbol', '(L')
|
||||
let close_lnum_symbol = get(g:, 'airline#extensions#languageclient#close_lnum_symbol', ')')
|
||||
|
||||
return open_lnum_symbol . linenumber_of_first_problem . close_lnum_symbol
|
||||
endfunction
|
||||
|
||||
function! airline#extensions#languageclient#get(type)
|
||||
let is_err = a:type == s:severity_error
|
||||
let symbol = is_err ? s:error_symbol : s:warning_symbol
|
||||
|
||||
let count = 0
|
||||
for d in s:diagnostics_for_buffer()
|
||||
if d.severity == a:type
|
||||
let count += 1
|
||||
endif
|
||||
endfor
|
||||
|
||||
if count == 0
|
||||
return ''
|
||||
endif
|
||||
|
||||
if s:show_line_numbers == 1
|
||||
return s:airline_languageclient_count(count, symbol) . <sid>airline_languageclient_get_line_number(a:type)
|
||||
else
|
||||
return s:airline_languageclient_count(count, symbol)
|
||||
endif
|
||||
endfunction
|
||||
|
||||
function! airline#extensions#languageclient#get_warning()
|
||||
return airline#extensions#languageclient#get(s:severity_warning)
|
||||
endfunction
|
||||
|
||||
function! airline#extensions#languageclient#get_error()
|
||||
return airline#extensions#languageclient#get(s:severity_error)
|
||||
endfunction
|
||||
|
||||
function! airline#extensions#languageclient#init(ext)
|
||||
call airline#parts#define_function('languageclient_error_count', 'airline#extensions#languageclient#get_error')
|
||||
call airline#parts#define_function('languageclient_warning_count', 'airline#extensions#languageclient#get_warning')
|
||||
augroup airline_languageclient
|
||||
autocmd!
|
||||
autocmd User LanguageClientDiagnosticsChanged call <sid>get_diagnostics()
|
||||
augroup END
|
||||
endfunction
|
@ -148,7 +148,8 @@ function! airline#init#bootstrap()
|
||||
call airline#parts#define_empty(['hunks', 'branch', 'obsession', 'tagbar',
|
||||
\ 'syntastic-warn', 'syntastic-err', 'eclim', 'whitespace','windowswap',
|
||||
\ 'ycm_error_count', 'ycm_warning_count', 'neomake_error_count',
|
||||
\ 'neomake_warning_count', 'ale_error_count', 'ale_warning_count'])
|
||||
\ 'neomake_warning_count', 'ale_error_count', 'ale_warning_count',
|
||||
\ 'languageclient_error_count', 'languageclient_warning_count'])
|
||||
call airline#parts#define_text('capslock', '')
|
||||
call airline#parts#define_text('gutentags', '')
|
||||
call airline#parts#define_text('grepper', '')
|
||||
@ -194,9 +195,9 @@ function! airline#init#sections()
|
||||
endif
|
||||
endif
|
||||
if !exists('g:airline_section_error')
|
||||
let g:airline_section_error = airline#section#create(['ycm_error_count', 'syntastic-err', 'eclim', 'neomake_error_count', 'ale_error_count'])
|
||||
let g:airline_section_error = airline#section#create(['ycm_error_count', 'syntastic-err', 'eclim', 'neomake_error_count', 'ale_error_count', 'languageclient_error_count'])
|
||||
endif
|
||||
if !exists('g:airline_section_warning')
|
||||
let g:airline_section_warning = airline#section#create(['ycm_warning_count', 'syntastic-warn', 'neomake_warning_count', 'ale_warning_count', 'whitespace'])
|
||||
let g:airline_section_warning = airline#section#create(['ycm_warning_count', 'syntastic-warn', 'neomake_warning_count', 'ale_warning_count', 'languageclient_warning_count', 'whitespace'])
|
||||
endif
|
||||
endfunction
|
||||
|
@ -313,8 +313,10 @@ section.
|
||||
let g:airline_section_x (tagbar, filetype, virtualenv)
|
||||
let g:airline_section_y (fileencoding, fileformat)
|
||||
let g:airline_section_z (percentage, line number, column number)
|
||||
let g:airline_section_error (ycm_error_count, syntastic-err, eclim)
|
||||
let g:airline_section_warning (ycm_warning_count, syntastic-warn, whitespace)
|
||||
let g:airline_section_error (ycm_error_count, syntastic-err, eclim,
|
||||
languageclient_error_count)
|
||||
let g:airline_section_warning (ycm_warning_count, syntastic-warn,
|
||||
languageclient_warning_count, whitespace)
|
||||
|
||||
" [*] This section needs at least the fugitive extension or else
|
||||
" it will remain empty
|
||||
@ -1199,6 +1201,27 @@ current mode (only works in terminals iTerm, AppleTerm and xterm)
|
||||
`\ "\<C-V>": g:airline#themes#{g:airline_theme}#palette.visual.airline_a[1],`
|
||||
`\ }`
|
||||
|
||||
------------------------------------- *airline-languageclient*
|
||||
LanguageClient <https://github.com/autozimu/LanguageClient-neovim>
|
||||
|
||||
* enable/disable LanguageClient integration >
|
||||
let g:airline#extensions#languageclient#enabled = 1
|
||||
|
||||
* languageclient error_symbol >
|
||||
let airline#extensions#languageclient#error_symbol = 'E:'
|
||||
<
|
||||
* languageclient warning_symbol >
|
||||
let airline#extensions#languageclient#warning_symbol = 'W:'
|
||||
|
||||
* languageclient show_line_numbers >
|
||||
let airline#extensions#languageclient#show_line_numbers = 1
|
||||
<
|
||||
* languageclient open_lnum_symbol >
|
||||
let airline#extensions#languageclient#open_lnum_symbol = '(L'
|
||||
<
|
||||
* languageclient close_lnum_symbol >
|
||||
let airline#extensions#languageclient#close_lnum_symbol = ')'
|
||||
|
||||
==============================================================================
|
||||
ADVANCED CUSTOMIZATION *airline-advanced-customization*
|
||||
|
||||
@ -1251,9 +1274,10 @@ Before is a list of parts that are predefined by vim-airline.
|
||||
And the following are defined for their respective extensions:
|
||||
|
||||
`ale_error_count` `ale_warning_count` `branch` `eclim` `hunks`
|
||||
`neomake_error_count` `neomake_warning_count` `obsession`
|
||||
`syntastic-warn` `syntastic-err` `tagbar` `whitespace`
|
||||
`windowswap` `ycm_error_count` `ycm_warning_count`
|
||||
`languageclient_error_count` `languageclient_warning_count`
|
||||
`neomake_error_count` `neomake_warning_count` `obsession` `syntastic-warn`
|
||||
`syntastic-err` `tagbar` `whitespace` `windowswap` `ycm_error_count`
|
||||
`ycm_warning_count`
|
||||
|
||||
------------------------------------- *airline-accents*
|
||||
Accents can be defined on any part, like so: >
|
||||
|
@ -74,6 +74,8 @@ describe 'init sections'
|
||||
Expect airline#parts#get('windowswap').raw == ''
|
||||
Expect airline#parts#get('ycm_error_count').raw == ''
|
||||
Expect airline#parts#get('ycm_warning_count').raw == ''
|
||||
Expect airline#parts#get('languageclient_error_count').raw == ''
|
||||
Expect airline#parts#get('languageclient_warning_count').raw == ''
|
||||
end
|
||||
end
|
||||
|
||||
|
Loading…
Reference in New Issue
Block a user